# 11์ฅ Programming ํ์ด
455p_1๋ฒ)
#include <stdio.h>
int main()
{
int i = 0;
char yes_or_no;
union student
{
char number[50];
char student_number[50];
char name[50];
char phone[50];
};
union student user[100];
printf("ํ์์ด์ญ๋๊น?: ");
scanf_s("%c", &yes_or_no);
if (yes_or_no == 'y') //ํ์์ผ ๊ฒฝ์ฐ
{
printf("์ฑํจ์ ์
๋ ฅํด ์ฃผ์ธ์: ");
rewind(stdin);
gets_s(user[i].name, 50);
printf("ํ๋ฒ์ ์
๋ ฅํด ์ฃผ์ธ์: ");
rewind(stdin);
gets_s(user[i].student_number, 50);
printf("์ ํ๋ฒํธ๋ฅผ ์
๋ ฅํด ์ฃผ์ธ์: ");
rewind(stdin);
gets_s(user[i].phone, 50);
}
else //ํ์ ์ ๋ถ์ด ์๋ ๊ฒฝ์ฐ
{
printf("์ฑํจ์ ์
๋ ฅํด ์ฃผ์ธ์: ");
rewind(stdin);
gets_s(user[i].name, 50);
printf("์ฃผ๋ฏผ๋ฑ๋ก ๋ฒํธ๋ฅผ ์
๋ ฅํด ์ฃผ์ธ์: ");
rewind(stdin);
gets_s(user[i].number, 50);
printf("์ ํ๋ฒํธ๋ฅผ ์
๋ ฅํด ์ฃผ์ธ์: ");
rewind(stdin);
gets_s(user[i].phone, 50);
}
return 0;
}
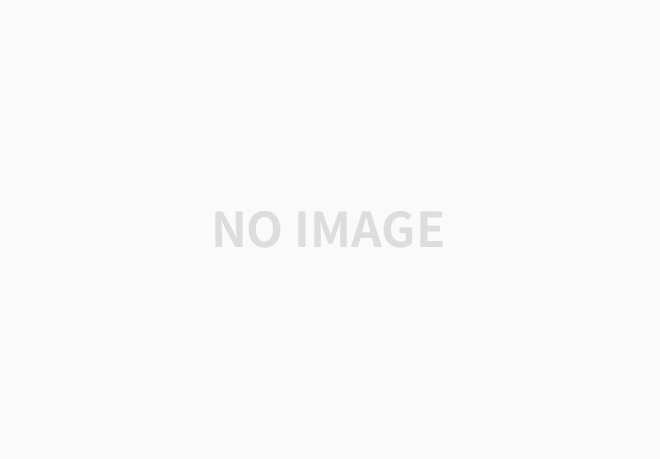
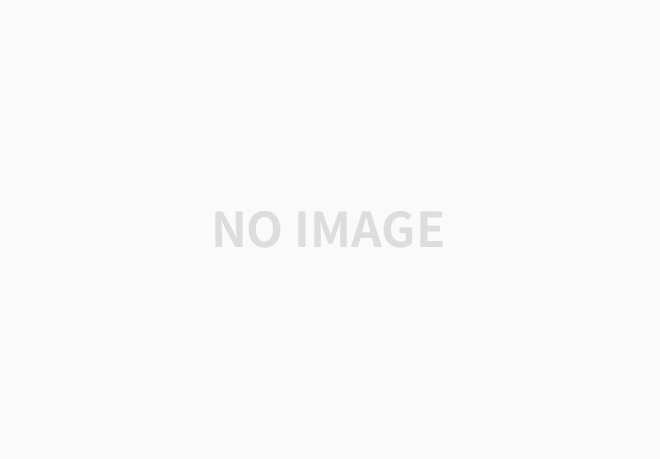
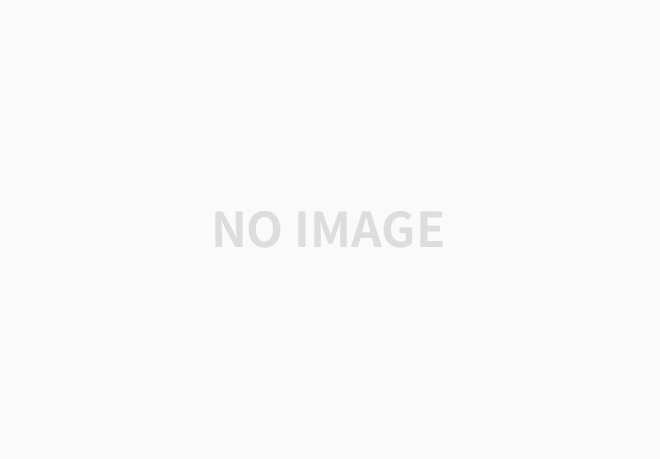
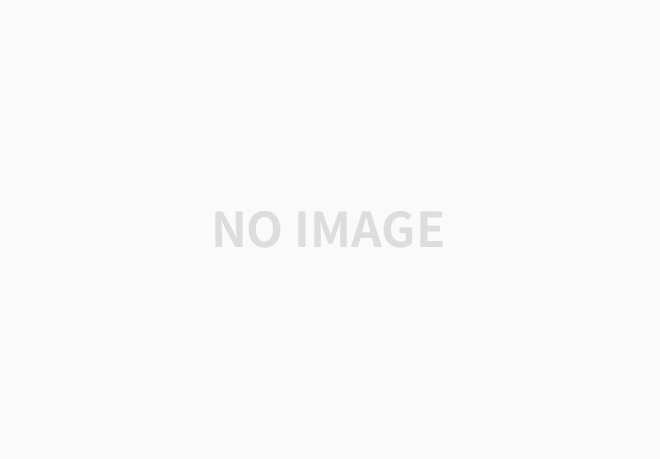
455p_2๋ฒ)
#include <stdio.h>
int main()
{
struct email
{
char sender[20];
char receiver[20];
char text[50];
char title[20];
char date[20];
};
struct email user =
{
"AA", "BB", "Let's meet at 7 pm on monday.", "Time to meet", "2021.02.04"
};
printf(" from. %s\n", user.sender);
printf("-ใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
ก-------------\n");
printf("| Title: %s |\n", user.title);
printf("| |\n");
printf("| %s |\n", user.text);
printf("| |\n");
printf("| %s|\n", user.date);
printf("-ใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
กใ
ก-------------\n");
printf(" To. %s\n", user.receiver);
return 0;
}
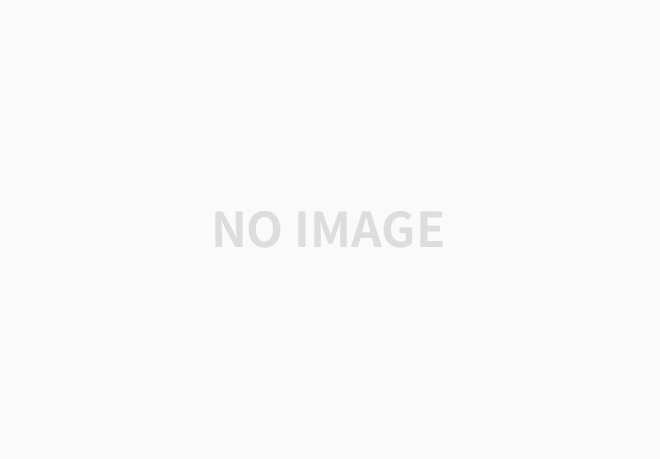
455p_3๋ฒ)
#include <stdio.h>
struct add
{
float x;
float y;
};
void calculate(struct add a, struct add b)
{
printf("%.1f + %.1fi\n", a.x + b.x, a.y + b.y);
}
int main()
{
struct add a;
struct add b;
printf("์ฒซ ๋ฒ์งธ ๋ณต์์๋ฅผ ์
๋ ฅํ์์ค(a,b):");
scanf_s("%f %f", &a.x, &a.y);
printf("๋ ๋ฒ์งธ ๋ณต์์๋ฅผ ์
๋ ฅํ์์ค(a,b):");
scanf_s("%f %f", &b.x, &b.y);
calculate(a, b);
return 0;
}
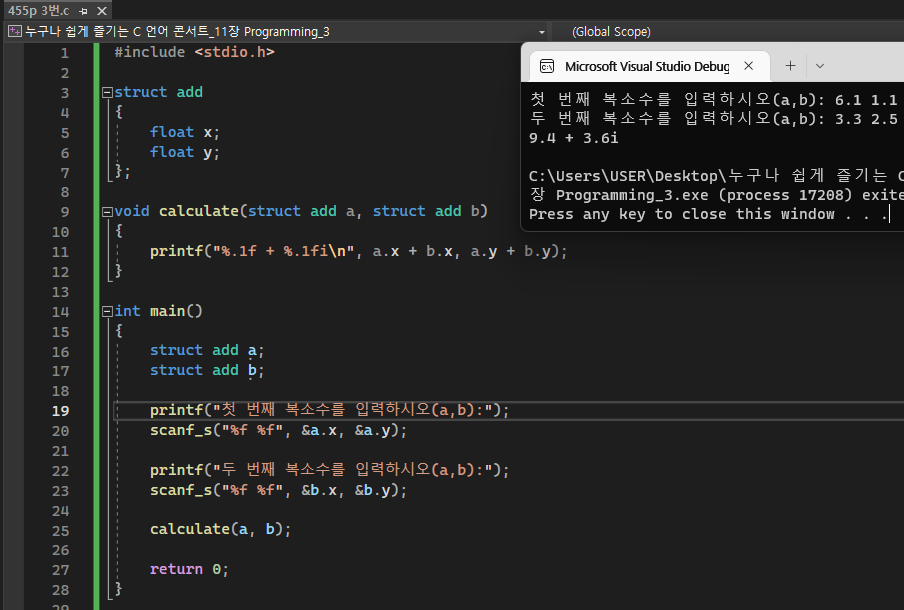
455p_4๋ฒ)
#include <stdio.h>
struct time
{
int hour;
int min;
int sec;
};
struct time start;
struct time end;
void diff_time(struct time start, struct time end)
{
printf("์์ ์๊ฐ: %d์๊ฐ %d๋ถ %d์ด\n", end.hour - start.hour, end.min - start.min, end.sec - start.sec);
}
int main()
{
printf("์์ ์๊ฐ: ");
scanf_s("%d %d %d", &start.hour, &start.min, &start.sec);
printf("์ข
๋ฃ ์๊ฐ: ");
scanf_s("%d %d %d", &end.hour, &end.min, &end.sec);
diff_time(start, end);
return 0;
}
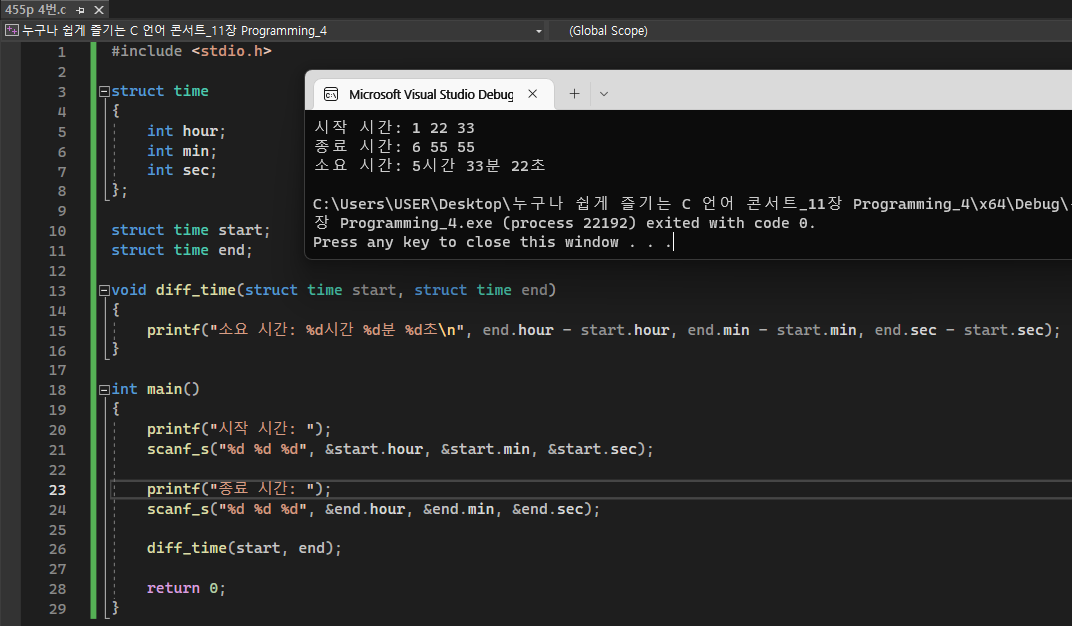
456p_5๋ฒ)
#include <stdio.h>
struct employee
{
int number;
char name[10];
char phone[20];
int age;
};
int main()
{
struct employee array[10] =
{
{ 1, "AA", "010-1111-1111", 20},
{ 2, "BB", "010-2222-2222", 22},
{ 3, "CC", "010-3333-3333", 33},
{ 4, "DD", "010-4444-4444", 44},
{ 5, "EE", "010-5555-5555", 45},
{ 6, "FF", "010-6666-6666", 30},
{ 7, "GG", "010-7777-7777", 77},
{ 8, "HH", "010-8888-8888", 37},
{ 9, "II", "010-9999-9999", 40},
{ 10, "JJ", "010-0000-0000", 41},
};
for (int i = 0; i < 10; i++)
{
if (array[i].age >= 20 && array[i].age <= 30)
{
printf("%d, %s, %s, %d\n", array[i].number, array[i].name, array[i].phone, array[i].age);
}
}
return 0;
}
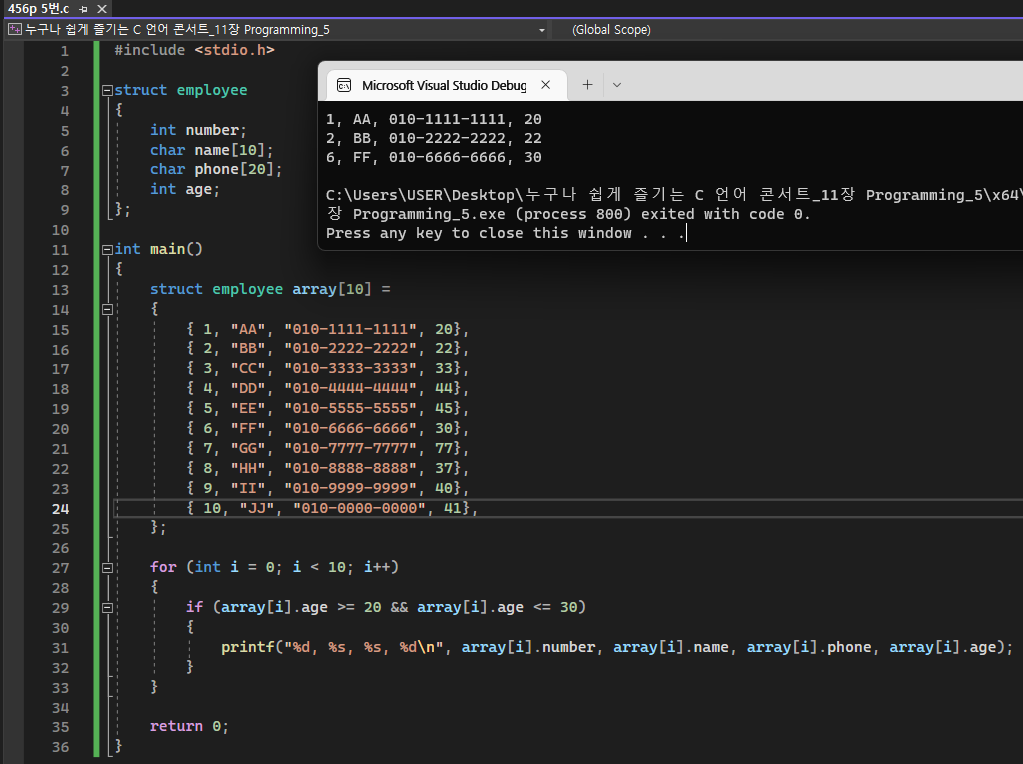
456p_6๋ฒ)
#include <stdio.h>
#include <string.h>
struct system
{
char name[10];
int price;
int num;
int total;
};
int main()
{
struct system A[100] =
{
{ "Apple", 1000, 3, 3000 },
{ "Banana", 1200, 2, 2400 },
{ "Plum", 2000, 5, 10000 }
};
char input[10];
printf("๊ฒ์ํ์ค ์ํ๋ช
์ ์
๋ ฅํด ์ฃผ์ธ์: ");
gets_s(input, 10);
for (int i = 0; i < 3; i++)
{
if (strcmp(input, A[i].name) == 0)
{
printf("=========================\n");
printf("์ํ๋ช
: %s\n", A[i].name);
printf("์ํ ๊ฐ๊ฒฉ: %d\n", A[i].price);
printf("์ํ ๊ฐ์: %d\n", A[i].num);
printf("=========================\n");
}
}
return 0;
}
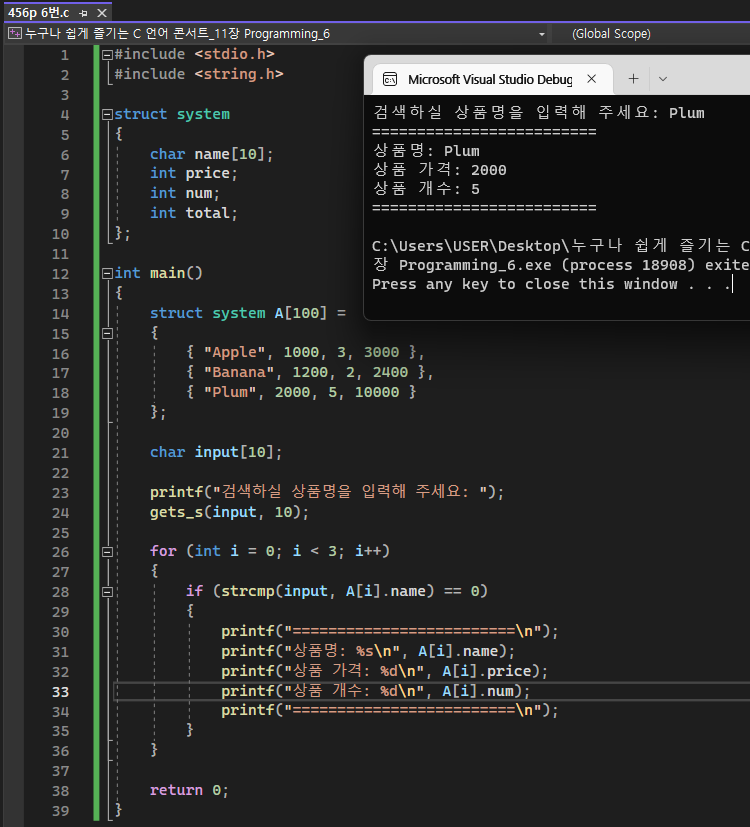
456p_7๋ฒ)
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
enum game
{
scissors, rock, paper
};
int main()
{
int user = 10;
enum game com;
srand(time(NULL));
com = rand() % 3;
printf("๊ฐ์(0), ๋ฐ์(1), ๋ณด(2)๋ฅผ ์
๋ ฅํ์ธ์: ");
scanf_s("%d", &user);
if (com == user)
{
printf("๋น๊ฒผ์ต๋๋ค.");
}
else if (user == rock)
{
if (com == paper)
{
printf("์ปดํจํฐ: %d, ์ฌ์ฉ์: %d\n", com, user);
printf("์ปดํจํฐ ์น๋ฆฌ\n");
}
else
{
printf("์ปดํจํฐ: %d, ์ฌ์ฉ์: %d\n", com, user);
printf("์ฌ์ฉ์ ์น๋ฆฌ\n");
}
}
else if (user == paper)
{
if (com == scissors)
{
printf("์ปดํจํฐ: %d, ์ฌ์ฉ์: %d\n", com, user);
printf("์ปดํจํฐ ์น๋ฆฌ\n");
}
else
{
printf("์ปดํจํฐ: %d, ์ฌ์ฉ์: %d\n", com, user);
printf("์ฌ์ฉ์ ์น๋ฆฌ\n");
}
}
else
{
if (com == rock)
{
printf("์ปดํจํฐ: %d, ์ฌ์ฉ์: %d\n", com, user);
printf("์ปดํจํฐ ์น๋ฆฌ\n");
}
else
{
printf("์ปดํจํฐ: %d, ์ฌ์ฉ์: %d\n", com, user);
printf("์ฌ์ฉ์ ์น๋ฆฌ\n");
}
}
return 0;
}
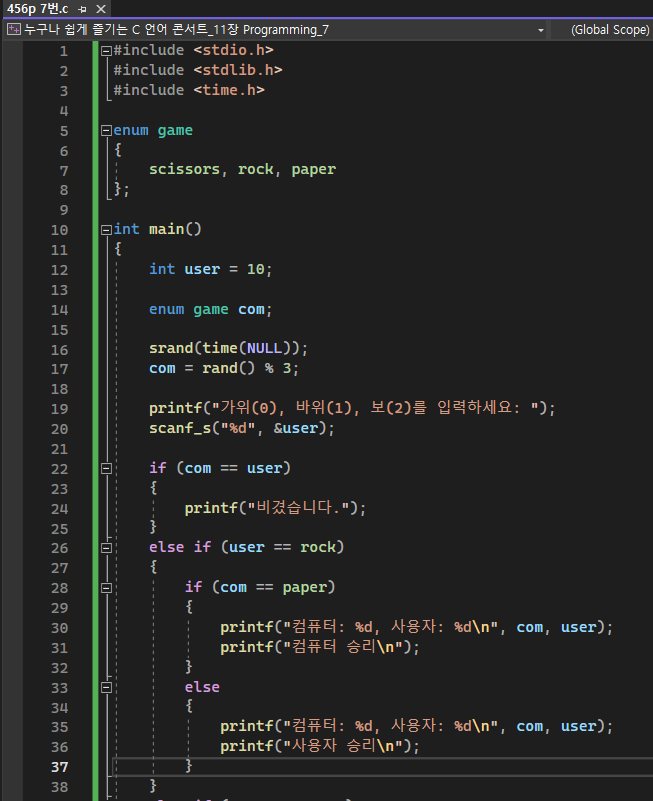
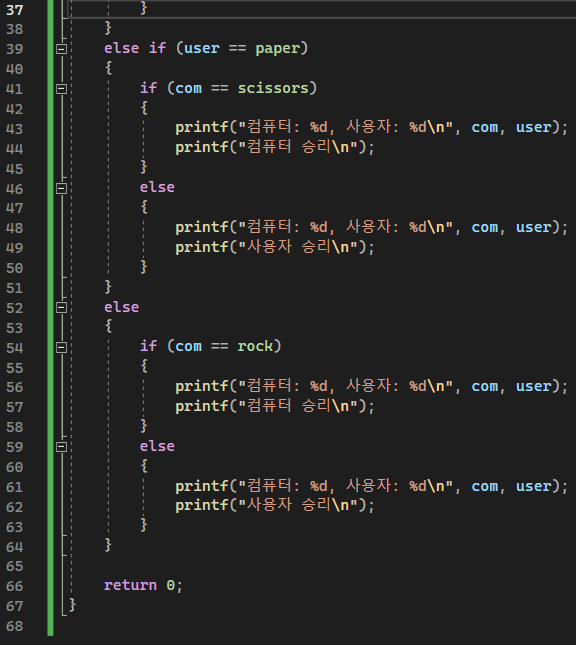
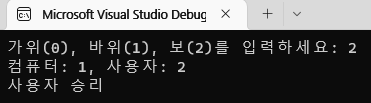